Last updated on January 9th, 2023 at 02:21 pm
In this tutorial we are going to create a button which animates after click. This can be used when you have an ajax / dynamic call to a different page and want user to wait with some interactive messaging. Similar to adding a “Loading image or spinner”
Let us start by adding a HTML Button
<input type="button" id="login_btn" value="Generate Backup Now" onclick=""/><br />
Add some style to the button to make it look neat
<style>
#login_btn {
background-color: #618862; /* Green */
border: 2px;
border-radius: 4px;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
}
#login_btn:hover {
background-color: #f44336;
color: white;
}
</style>
Now you will get a button like this
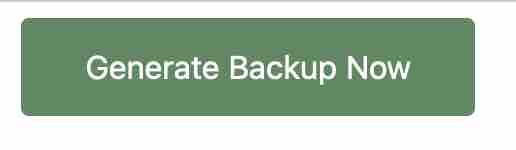
Next step is to Animate the button when user click on it. For that load the jquery
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script>
Add a click function which basically calls another function known as startAnimation()
$('#login_btn').click(function() {
var i=0;
startAnimation(i);
});
function startAnimation(i) {
document.getElementById('login_btn').disabled = 'true';
var b = [".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............",".", "..", "...", "....", ".....","......",".......","........",".........","..........","...........","............",".............","Backup Generated" ];
var a = setInterval(function () {
console.log(i)
if(b[i]=="Backup Generated")
{
clearInterval(a);
$("#login_btn").val("Backup Generation Completed")
}
else
{
$("#login_btn").val("Generating Backup " + b[i++]);
}
}, 500);
}
});
In the above code in a nutshell we are basically
- Disabling the button
- Add some animation with dots (….)
- Loop the content by using setInterval, every 500 ms
- When array value is “Backup Generated” the interval is cleared